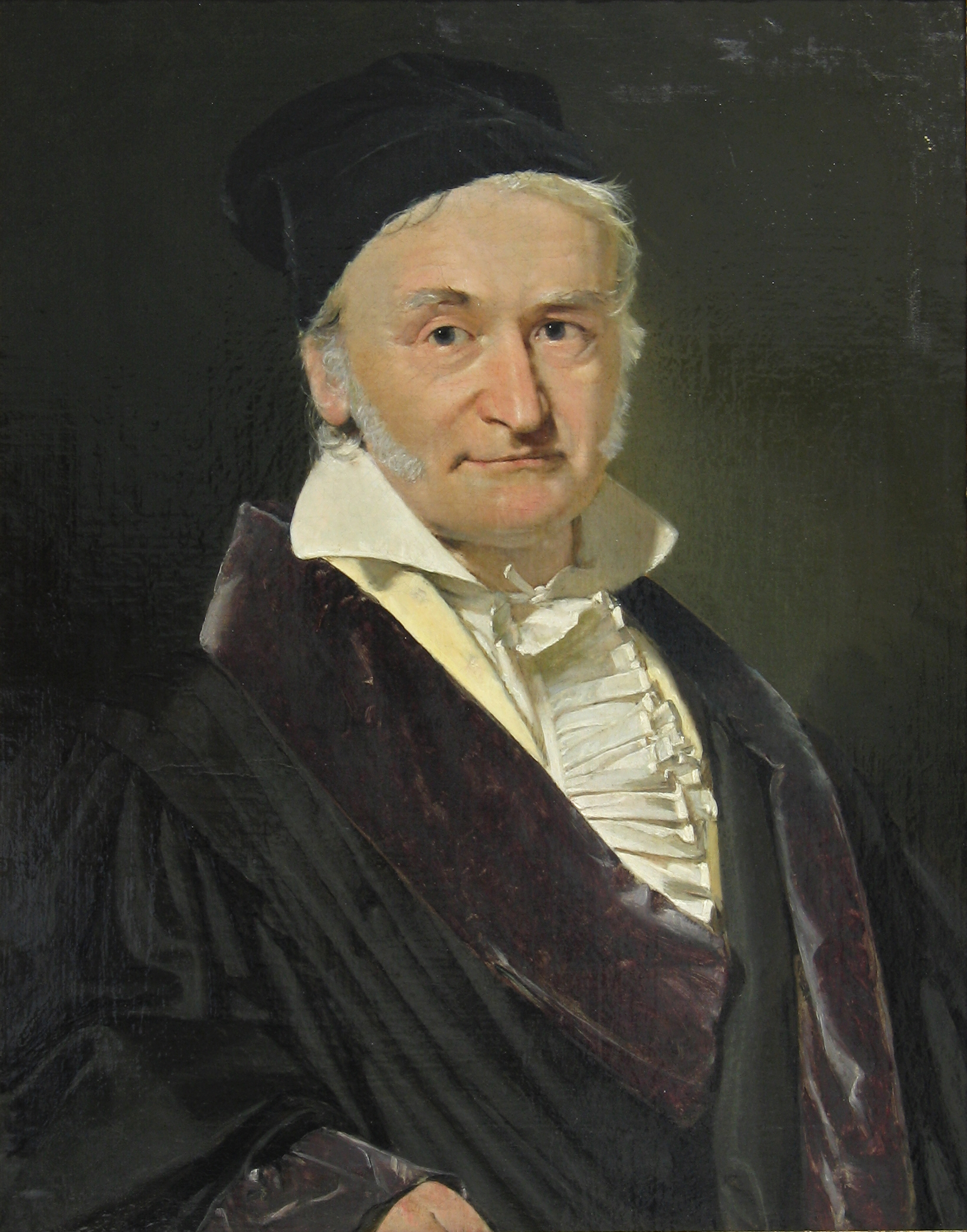
The Bisection Method, also known as the Binary Search Method for root-finding, is one of the oldest and most straightforward numerical methods. Its origins can be traced back to ancient mathematics, but it was first formally described by Bolzano in his 1817 paper on the Intermediate Value Theorem. Despite its simplicity, the method remains valuable today due to its reliability and guaranteed convergence for continuous functions.