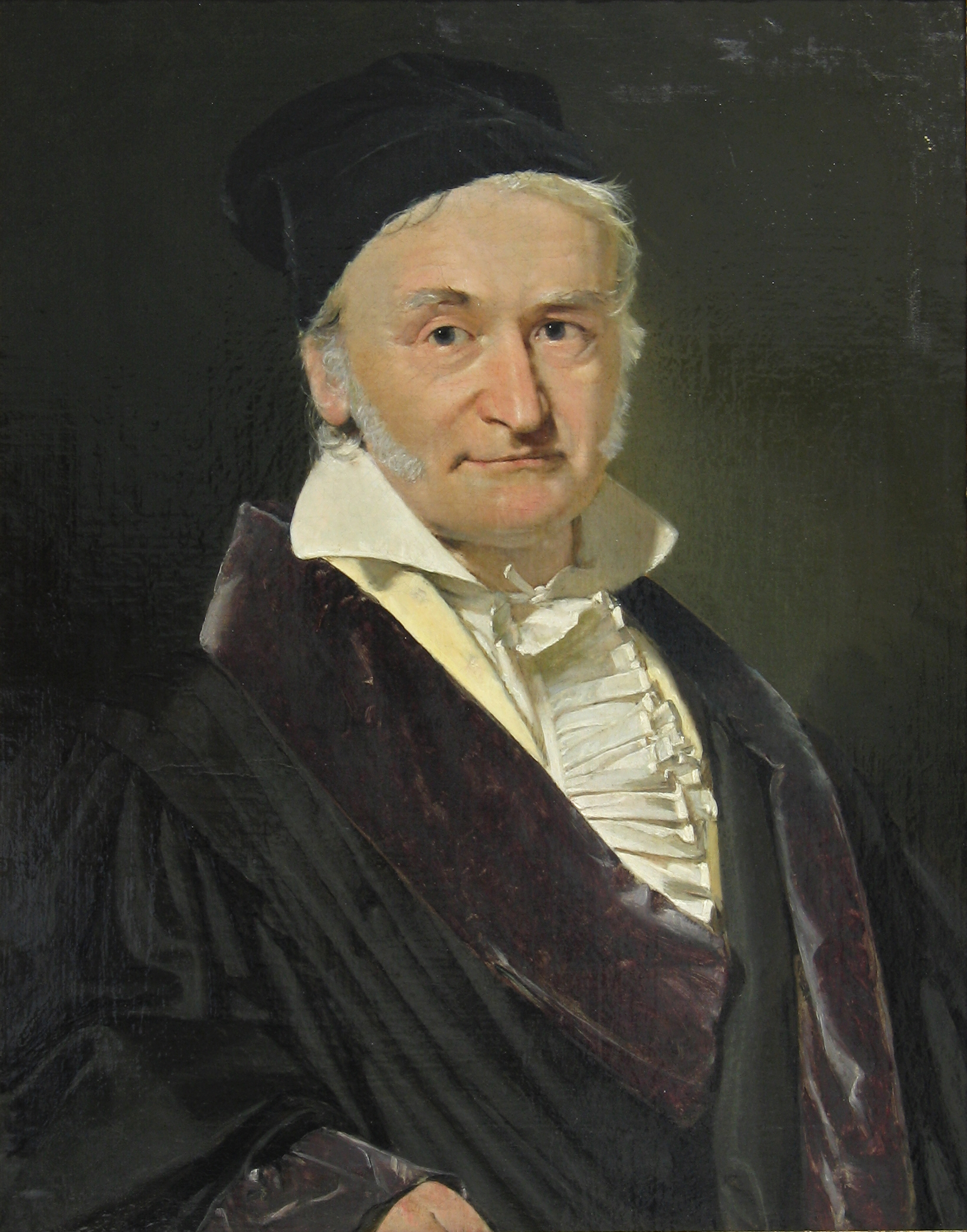
The Fast Fourier Transform has a rich history dating back to 1805 when Carl Friedrich Gauss first developed an algorithm for calculating the coefficients of a finite Fourier series. However, the modern FFT algorithm was popularized by James Cooley and John Tukey in their 1965 paper. Their algorithm, known as the Cooley-Tukey FFT algorithm, revolutionized digital signal processing by making Fourier analysis practical for computers. The algorithm was actually rediscovered, as Gauss's earlier work wasn't widely known. The development of the FFT is considered one of the most important algorithmic advances of the 20th century.