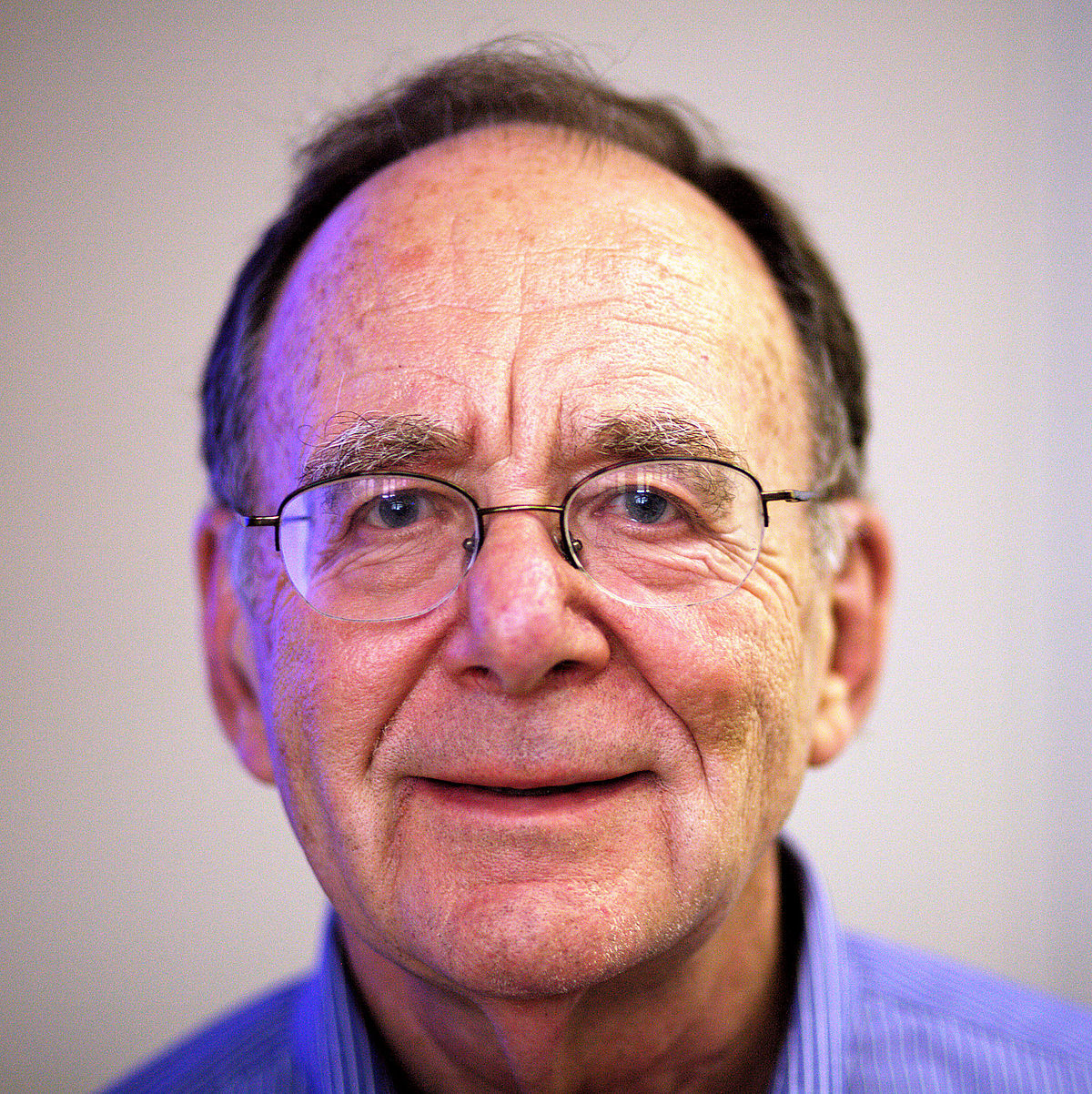
The Rabin-Karp algorithm was developed by Richard M. Karp and Michael O. Rabin in 1987. It combines two key ideas: rolling hash functions and string matching. The algorithm was a significant improvement over naive string matching for specific use cases, particularly in detecting plagiarism by finding duplicate content in large texts. The use of rolling hash functions made it possible to update hash values efficiently, leading to its widespread adoption in document similarity detection and bioinformatics applications.